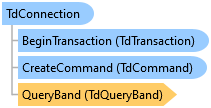
'Declaration Public NotInheritable Class TdConnection Inherits System.Data.Common.DbConnection Implements System.ComponentModel.IComponent, System.Data.IDbConnection, System.ICloneable, System.IDisposable
'Usage Dim instance As TdConnection
public sealed class TdConnection : System.Data.Common.DbConnection, System.ComponentModel.IComponent, System.Data.IDbConnection, System.ICloneable, System.IDisposable
public ref class TdConnection sealed : public System.Data.Common.DbConnection, System.ComponentModel.IComponent, System.Data.IDbConnection, System.ICloneable, System.IDisposable
TdConnection is a Component and therefore implements the IDisposable interface. It is highly recommended that TdConnection.Dispose or TdConnection.Close be called before an instance of this class is released for garbage collection. Garbage collection is not deterministic and therefore valuable Teradata resources (i.e. session) might not be released immediately. Also TdConnection.Dispose or TdConnection.Close returns the Teradata session back to the pool if connection pooling is enabled. See TdConnection.ConnectionString for details on connection pooling.
TdConnection also allows for explicit transactions via the TdConnection.BeginTransaction method.
class Example { static void Main(String[] args) { TdConnection cn = null; try { cn = new TdConnection("Data Source=Teradata1;User Id=ab;Password=ab;"); cn.Open(); TdCommand cmd = new TdCommand("Show Table Customers", cn); String customers = (String) cmd.ExecuteScalar(); cmd.Dispose(); } catch(TdException e) { Debug.WriteLine(e.Message); } finally { if (null != cn) { cn.Close(); } } } }
System.Object
System.MarshalByRefObject
System.ComponentModel.Component
System.Data.Common.DbConnection
Teradata.Client.Provider.TdConnection
Target Platforms: Windows 8.1, Windows 10, Windows Server 2012 R2, Windows Server 2016, Windows Server 2019