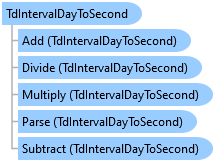
'Declaration Public Structure TdIntervalDayToSecond Inherits System.ValueType Implements System.Data.SqlTypes.INullable, System.IComparable, System.IComparable(Of TdIntervalDayToSecond), System.IConvertible, System.IEquatable(Of TdIntervalDayToSecond), System.IFormattable, System.Xml.Serialization.IXmlSerializable
'Usage Dim instance As TdIntervalDayToSecond
public struct TdIntervalDayToSecond : System.ValueType, System.Data.SqlTypes.INullable, System.IComparable, System.IComparable<TdIntervalDayToSecond>, System.IConvertible, System.IEquatable<TdIntervalDayToSecond>, System.IFormattable, System.Xml.Serialization.IXmlSerializable
public value class TdIntervalDayToSecond : public System.ValueType, System.Data.SqlTypes.INullable, System.IComparable, System.IComparable<TdIntervalDayToSecond>, System.IConvertible, System.IEquatable<TdIntervalDayToSecond>, System.IFormattable, System.Xml.Serialization.IXmlSerializable
TdIntervalDayToSecond is a .NET Data Provider for Teradata specific type, designed to support a SQL Interval Day To Second data type.
The TdIntervalDayToSecond supports the Teradata Interval Day (precision) To Second (scale) data type where precision indicates the number of digits in the day (from 1 - 4) and scale indicates the fractional precision for the values of seconds. The scale may range from 0 to 6.
The interval value must be specified in the following format :
[sign][days][space character][hh]:[mm]:[ss].[ffffff]
Below is the description of each format item.
Format Item | Description |
---|---|
sign | Optional - . Defaults as space character (+). |
days | Required number of days (one to four digits in length). |
hh | Required number of hours from 00 - 23. |
mm | Required number of minutes from 00 - 59. |
ss | Required number of seconds from 00 - 59. |
ffffff | Optionally the number of fractional seconds (0 to 6 digits in length). |
The .Net Framework does not have a system type that directly corresponds to the SQL Interval Day To Second data type. The .NET Data Provider for Teradata Version 13.0 version and earlier versions map Interval Day To Second to System.String. With version 13.1 of the provider, TdIntervalDayToSecond is available to retrieve and manipulate data of type Interval Day To Second.
The range of values for the TdIntervalDayToSecond containing a day precision of 1 is as follows:
Day Precision | Second Scale |
Minimum |
Maximum |
---|---|---|---|
1 | 0 |
-'9 23:59:59' |
'9 23:59:59' |
1 | 1 |
-'9 23:59:59.9' |
'9 23:59:59.9' |
1 | 2 |
-'9 23:59:59.99' |
'9 23:59:59.99' |
1 | 3 |
-'9 23:59:59.999' |
'9 23:59:59.999' |
1 | 4 |
-'9 23:59:59.9999' |
'9 23:59:59.9999' |
1 | 5 |
-'9 23:59:59.99999' |
'9 23:59:59.99999' |
1 | 6 |
-'9 23:59:59.999999' |
'9 23:59:59.999999' |
The range of values for day precision values of 2,3 and 4 all follow the same pattern. For completeness, the following chart displays the range of values for a TdIntervalDayToSecond with a day precision of 4.
Day Precision |
Second Scale |
Minimum |
Maximum |
---|---|---|---|
4 | 0 |
-'9999 23:59:59' |
'9999 23:59:59' |
4 | 1 |
-'9999 23:59:59.9' |
'9999 23:59:59.9' |
4 | 2 |
-'9999 23:59:59.99' |
'9999 23:59:59.99' |
4 | 3 |
-'9999 23:59:59.999' |
'9999 23:59:59.999' |
4 | 4 |
-'9999 23:59:59.9999' |
'9999 23:59:59.9999' |
4 | 5 |
-'9999 23:59:59.99999' |
'9999 23:59:59.99999' |
4 | 6 |
-'9999 23:59:59.999999' |
'9999 23:59:59.999999' |
TdIntervalDayToSecond also supports TdIntervalDayToSecond.Null. This is a very important feature. An application is no longer required to call TdDataReader.IsDBNull before invoking the corresponding TdDataReader "Get" method. This will improve overall performance.
A TdIntervalDayToSecond structure allows arithmetic, comparision and conversion operations to be performed.
A TdIntervalDayToSecond may also be specified as an in, out, or in/out parameter to a stored procedure. In order to maintain backward compatibility with previous versions of the provider (release 13.0 and prior), a Connection String Attribute TdConnectionStringBuilder.EnableTdIntervals has been added. When the EnableTdIntervals attribute is true
, TdParameter.ProviderSpecificValue will return the data as the provider specific type of TdIntervalDayToSecond. When EnableTdIntervals is false
, TdParameter.ProviderSpecificValue will return the data as a .NET Framework Library data type of String.
The TdParameter.Value will also return the .NET Framework Library data type of System.String when EnableTdIntervals is false
to maintain backward compatibility. The TdParameter.Value will return a .NET Framework data type of TimeSpan when EnableTdIntervals is true
.
For more information on the Teradata Interval Day To Second data type please see the 'SQL Data Types and Literals'.
The following example shows how to retrieve a TdIntervalDayToSecond, modify the interval, and then update the table.
Public void IntervalExample(TdCommand cmd, String model) { cmd.Parameters.Clear(); cmd.CommandText = "SELECT StartDate, LeaseReturn, DelayPeriod " + "FROM AutoLeases " + "WHERE Model = ?"; cmd.CommandType = CommandType.Text; cmd.Parameters.Add(null, TdType.VarChar, 10, ParameterDirection.Input, true, 0, 0, null, DataRowVersion.Default, model); Int32 row = 0; TdDate [] startDate; TdTimestamp [] leaseReturn; TdIntervalDayToSecond [] leaseLen; using (TdDataReader dr = cmd.ExecuteReader()) { startDate = new TdDate[dr.RecordsReturned]; leaseReturn = new TdTimestamp[dr.RecordsReturned]; leaseLen = new TdIntervalDayToSecond [dr.RecordsReturned]; // Specifying an interval of 7 days, 1 hour, 30 minutes, 0 seconds, 0 fractional seconds // with a day precision of 4 and 0 scale TdIntervalDayToSecond leaseReturnExtension = new TdIntervalDayToSecond(7, 1, 30, 0, 0, 4, 0); while (dr.Read()) { // Retrieving the dates startDate[row] = dr.GetTdDate(0); leaseReturn[row] = dr.GetTimestamp(1); leaseLen[row] = dr.GetTdIntervalDayToSecond(2); // Adding extension to the lease return leaseReturn[row] = leaseReturn[row] + leaseReturnExtension; // Adding extension to the lease length; leaseLen[row] = leaseLen[row] + leaseReturnExtension; row++; } } cmd.Parameters.Clear(); cmd.CommandText = "UPDATE AutoLeases " + "SET LeaseReturn = ?, LeaseLen = ? " + "WHERE Model = ?"; cmd.Parameters.Add(null, TdType.Timestamp, 0, ParameterDirection.Input, true, 0, 0, null, DataRowVersion.Default, null); cmd.Parameters.Add(null, TdType.IntervalDayToSecond, 0, ParameterDirection.Input, true, 0, 0, null, DataRowVersion.Default, null); cmd.Parameters.Add(null, TdType.VarChar, 9, ParameterDirection.Input, true, 0, 0, null, DataRowVersion.Default, model); row--; while(row >= 0) { cmd.Parameters[0].Value = leaseReturn[row]; cmd.Parameters[1].Value = leaseLen[row]; cmd.Parameters[2].Value = model; cmd.ExecuteNonQuery(); row--; } }
System.Object
System.ValueType
Teradata.Client.Provider.TdIntervalDayToSecond
Target Platforms: Windows 8.1, Windows 10, Windows Server 2012 R2, Windows Server 2016, Windows Server 2019
TdIntervalDayToSecond Members
Teradata.Client.Provider Namespace
EnableTdIntervals Property
Interval Connection String Attribute
Enabling Provider Specific Types
Provider Specific Type: Interval Type Overview